1.한문자씩 읽고, 한문자씩 쓰기
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdlib.h> | |
#include <stdio.h> | |
int main(void) { | |
//파일 포인터 변수 | |
FILE *rfp, *wfp; | |
int c; | |
//파일 읽기 전용으로 열기 | |
if ((rfp = fopen("unix2.txt", "r")) == NULL) { | |
perror("fopen: unix.txt"); | |
exit(1); | |
} | |
//파일을 쓰기 전용으로 열기 | |
if ((wfp = fopen("aaaa.txt", "w")) == NULL) { | |
perror("fopen: unix.out"); | |
exit(1); | |
} | |
//fgetc 함수를 이용해서 wfp 파일 포인터에 unix.txt 내용을 쓰기 | |
//fgetc 함수는 문자 하나씩 읽는 함수. | |
while ((c = fgetc(rfp)) != EOF) { | |
fputc(c, wfp); | |
} | |
fclose(rfp); | |
fclose(wfp); | |
return 0; | |
} | |
//참고 | |
//http://blog.naver.com/PostView.nhn?blogId=highkrs&logNo=220195201545&parentCategoryNo=&categoryNo=&viewDate=&isShowPopularPosts=false&from=postView |
결과
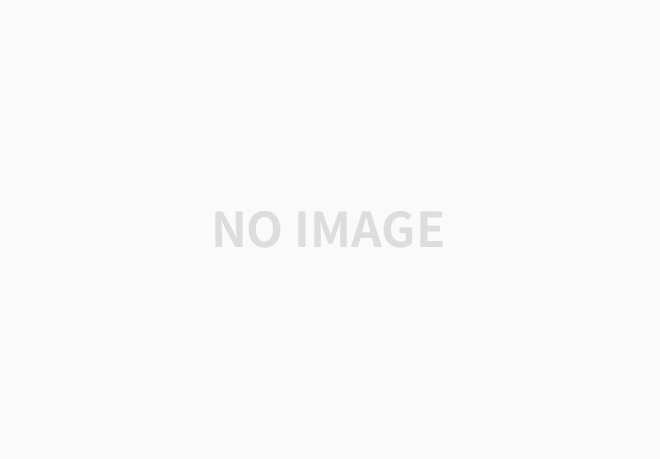
2.한문자열씩 읽고 쓰기
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdlib.h> | |
#include <stdio.h> | |
/* | |
fgets : 개행 문자를 만나거나 max-1 개를 읽으면 마지막에 종료 문자를 추가합니다. | |
gets에서는 개행 문자를 지우고 종료 문자를 추가하지만 fgets에서는 개행 문자도 그대로 읽습니다. | |
*/ | |
int main(void) { | |
//파일 포인터 변수 | |
FILE *rfp, *wfp; | |
char buf[BUFSIZ]; | |
//파일 읽기 전용으로 열기 | |
if ((rfp = fopen("unix2.txt", "r")) == NULL) { | |
perror("fopen: unix.txt"); | |
exit(1); | |
} | |
//unix6.txt 에 붙이기 | |
if ((wfp = fopen("unix6.txt", "a")) == NULL) { | |
perror("fopen: unix6.txt"); | |
exit(1); | |
} | |
//fgets :파일 스트림의 버퍼에서 문자열을 읽는 함수 | |
//buffer 문자열을 기록할 버퍼 | |
//BUFSIZ 읽어올 문자열을 구성하는 문자 최대 개수 -1 | |
//rfp 입력 파일 스트림 | |
while (fgets(buf, BUFSIZ, rfp) != NULL) { | |
fputs(buf, wfp); | |
} | |
//파일 디스크립터를 닫는다. | |
fclose(rfp); | |
fclose(wfp); | |
return 0; | |
} | |
//참고 | |
//http://blog.naver.com/PostView.nhn?blogId=highkrs&logNo=220195938896&parentCategoryNo=&categoryNo=&viewDate=&isShowPopularPosts=false&from=postView | |
//http://ehpub.co.kr/fputs-%ed%95%a8%ec%88%98/ |
결과
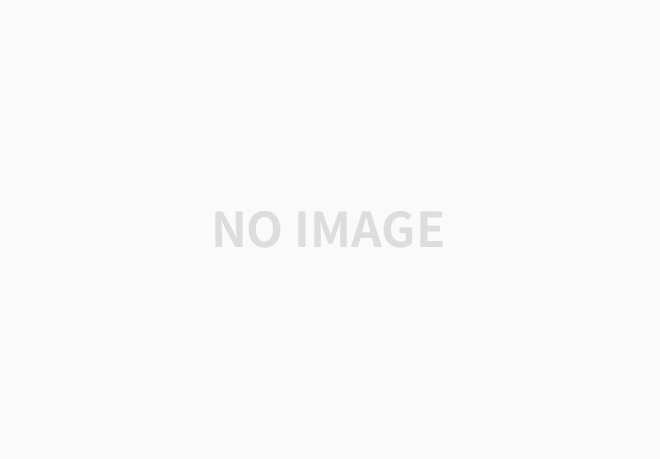
3.fread 함수
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdlib.h> | |
#include <stdio.h> | |
int main(void) { | |
FILE *rfp; | |
char buf[BUFSIZ]; | |
int n; | |
//읽기전용으로 파일을 열고 | |
if ((rfp = fopen("unix77.txt", "r")) == NULL) { | |
perror("fopen: unix77.txt"); | |
exit(1); | |
} | |
//fread : 스트림에서 데이터 블록을 읽어온다. 스트림에서 3 개의 원소를 가지는 배열을 읽어온다. | |
// 이 때, 각 원소의 크기는 sizeof(char)*2 바이트 이고 buf가 가리키는 배열에 넣게 된다. | |
//리턴값은 읽어들인 바이트 수 | |
while ((n=fread(buf, sizeof(char)*2, 3, rfp)) > 0) { | |
buf[6] = '\0'; | |
printf("n=%d, buf=%s\n", n, buf); //n=2, buf=abcde | |
} | |
fclose(rfp); | |
return 0; | |
} |
결과
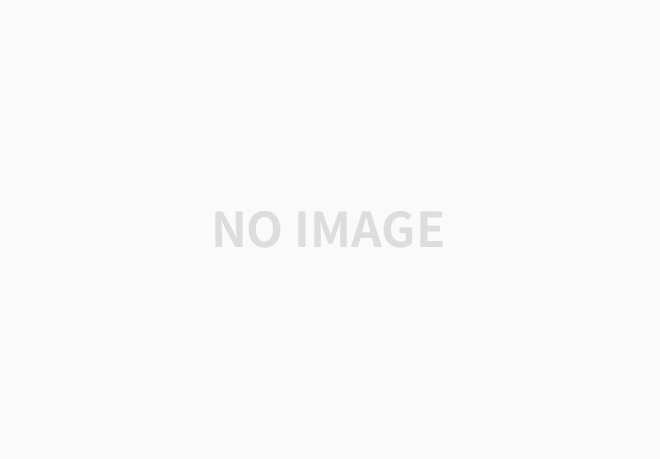
4.fwrite 함수
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdlib.h> | |
#include <stdio.h> | |
int main(void) { | |
FILE *rfp, *wfp; | |
char buf[BUFSIZ]; | |
int n; | |
//파일 읽기전용으로 열기 | |
if ((rfp = fopen("unix77.txt", "r")) == NULL) { | |
perror("fopen: unix77.txt"); | |
exit(1); | |
} | |
//unix88에 계속 붙여넣기 | |
if ((wfp = fopen("unix88.txt", "a")) == NULL) { | |
perror("fopen: unix88.txt"); | |
exit(1); | |
} | |
while ((n = fread(buf, sizeof(char)*2, 3, rfp)) > 0) { | |
//위에서 fread 가 (읽은바이트 수 * sizeof(char) *2) 만큼 wfp 스트림에 쓴다. | |
fwrite(buf, sizeof(char)*2, n, wfp); | |
} | |
//파일 디스크립터 닫기 | |
fclose(rfp); | |
fclose(wfp); | |
return 0; | |
} |
결과
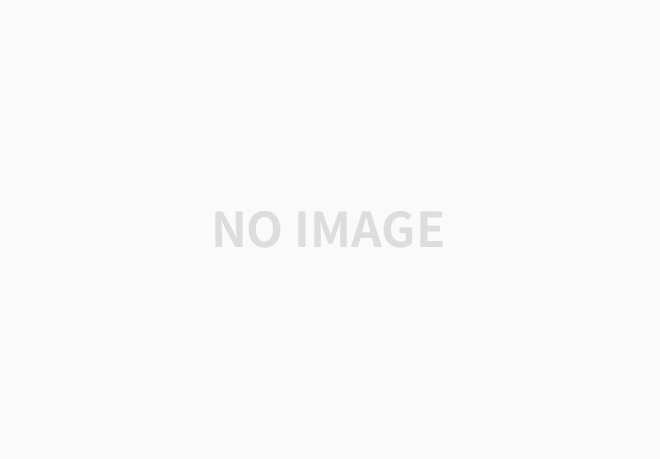
5.형식기반 출력함수
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdlib.h> | |
#include <stdio.h> | |
//형식기반 파일 입력 | |
int main(void) { | |
FILE *rfp; | |
int id, s1, s2, s3, s4, n; | |
if ((rfp = fopen("score.txt", "r")) == NULL) { | |
perror("fopen: score.txt"); | |
exit(1); | |
} | |
printf("id avg\n"); | |
//서식을 지정해서 문자열 읽기 | |
//성공하면 읽어온 값의 개수를 반환, 실패하면 EOF(-1)를 반환 | |
while ((n=fscanf(rfp, "%d %d %d %d %d", &id,&s1,&s2,&s3,&s4)) != EOF) { | |
printf("%d : %d\n", id, (s1+s2+s3+s4)/4); | |
} | |
fclose(rfp); | |
return 0; | |
} | |
//참고 | |
//http://blog.naver.com/PostView.nhn?blogId=sharonichoya&logNo=220502134809 |
결과
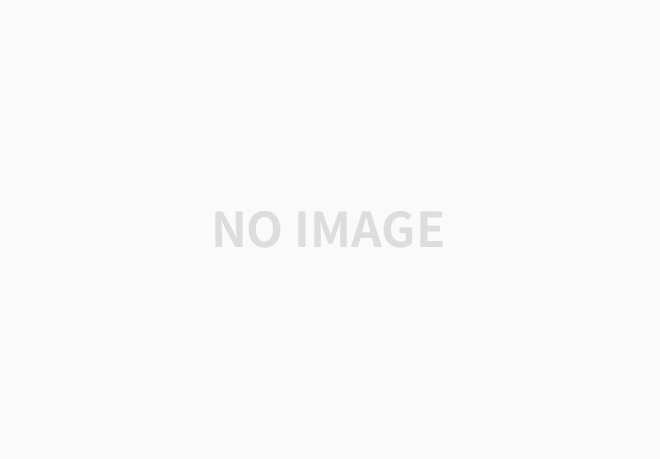
6.서식을 지정해서 파일에 문자열 쓰기
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdlib.h> | |
#include <stdio.h> | |
int main(void) { | |
FILE *rfp, *wfp; | |
int id, s1, s2, s3, s4, n; | |
if ((rfp = fopen("score.txt", "r")) == NULL) { | |
perror("fopen: score.txt"); | |
exit(1); | |
} | |
if ((wfp = fopen("myscore.txt", "w")) == NULL) { | |
perror("fopen: myscore.txt"); | |
exit(1); | |
} | |
//서식을 지정해서 파일에 문자열 쓰기 | |
fprintf(wfp, "id avg\n"); | |
while ((n=fscanf(rfp, "%d %d %d %d %d", &id,&s1,&s2,&s3,&s4)) != EOF) { | |
//지정한 파일에 출력 | |
fprintf(wfp, "%d : %d\n", id, (s1+s2+s3+s4)/4); | |
} | |
//파일 디스크립터 닫기 | |
fclose(rfp); | |
fclose(wfp); | |
return 0; | |
} |
결과
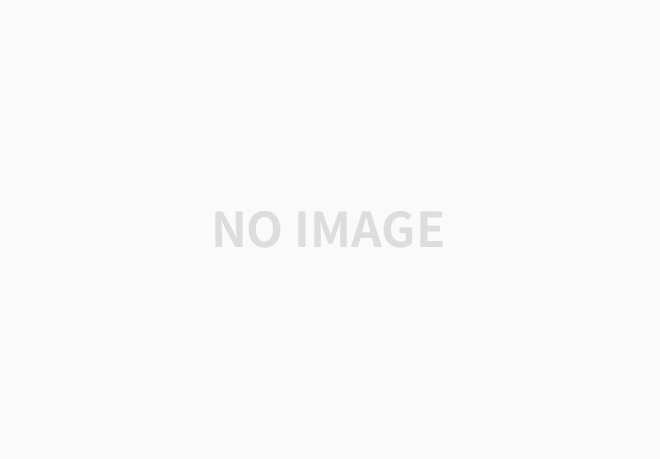
7.파일 오프셋
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <stdlib.h> | |
#include <stdio.h> | |
/* | |
fseek(파일포인터, 이동할크기, 기준점); | |
int fseek(FILE *_Stream, long _Offset, int _Origin); | |
성공하면 0, 실패하면 -1을 반환 | |
ftell(파일포인터); | |
long ftell(FILE *_Stream); | |
파일 포인터의 현재 위치를 반환, 실패하면 -1을 반환 | |
*/ | |
int main(void) { | |
int size; | |
FILE *fp = fopen("unix2.txt", "r"); // hello.txt 파일을 읽기 모드(r)로 열기. | |
// 파일 포인터를 반환 | |
fseek(fp, 0, SEEK_END); // 파일 포인터를 파일의 끝으로 이동시킴 | |
size = ftell(fp); // 파일 포인터의 현재 위치를 얻음 | |
printf("%d\n", size); //23 | |
fclose(fp); | |
return 0; | |
} |
결과
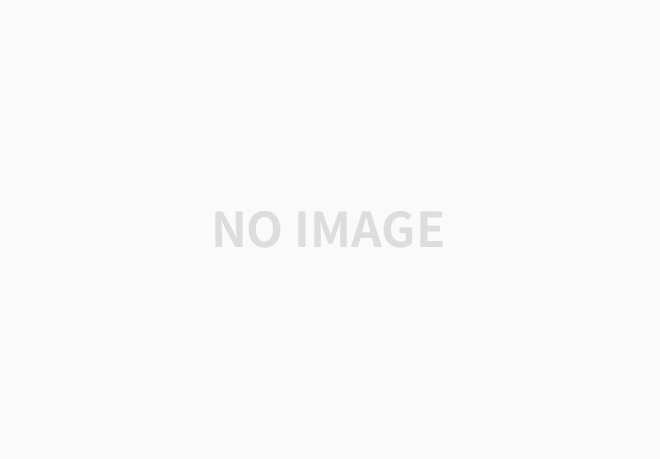
8.파일 기술자와 파일 포인터 간 변화
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <fcntl.h> | |
#include <stdlib.h> | |
#include <stdio.h> | |
int main(void) { | |
//파일 포인터 | |
FILE *fp; | |
//파일 디스크립터 | |
int fd; | |
//파일에서 읽은 값 담을 변수 | |
char str[BUFSIZ]; | |
//저수준 파일 입출력 함수로 파일 열기 | |
fd = open("unix2.txt", O_RDONLY); | |
if (fd == -1) { | |
perror("open"); | |
exit(1); | |
} | |
//fdopen 함수를 이용해서 파일 포인터 생성 | |
fp = fdopen(fd, "r"); | |
//위에서 생성한 파일 포인터를 통해서 고수준 파일 입출력 함수로 데이터를 읽는다. | |
fgets(str, BUFSIZ, fp); | |
printf("Read : %s\n", str); | |
//파일 포인터 닫기 | |
fclose(fp); | |
return 0; | |
} |
결과
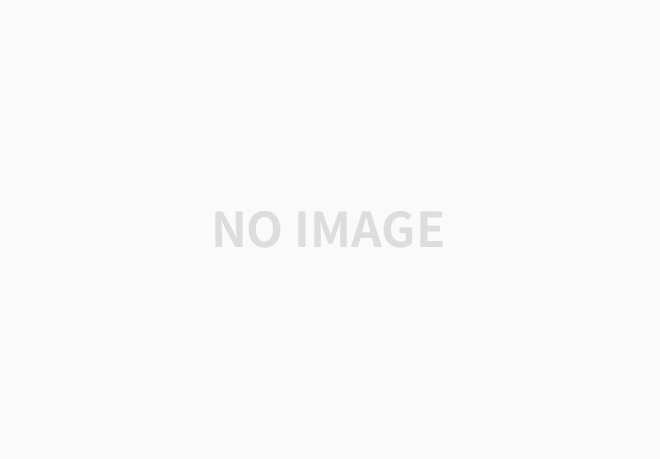
9.fileno 함수 사용하기
예제
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
#include <unistd.h> | |
#include <fcntl.h> | |
#include <stdlib.h> | |
#include <stdio.h> | |
int main(void) { | |
FILE *fp; | |
int fd, n; | |
char str[BUFSIZ]; | |
//고수준 파일 열기 함수로 읽기전용으로 파일 열기 | |
fp = fopen("unix2.txt", "r"); | |
if (fp == NULL) { | |
perror("fopen"); | |
exit(1); | |
} | |
//fileno 함수 인자로 위에서 파일을 열고 리턴 받은 파일 포인터를 전달 | |
fd = fileno(fp); | |
printf("fd : %d\n", fd); //3 | |
n = read(fd, str, BUFSIZ); | |
str[n] = '\0'; | |
printf("Read : %s\n", str); | |
//Read : unix system programing | |
//저수준 파일 디스크립터 닫기 | |
close(fd); | |
return 0; | |
} |
결과
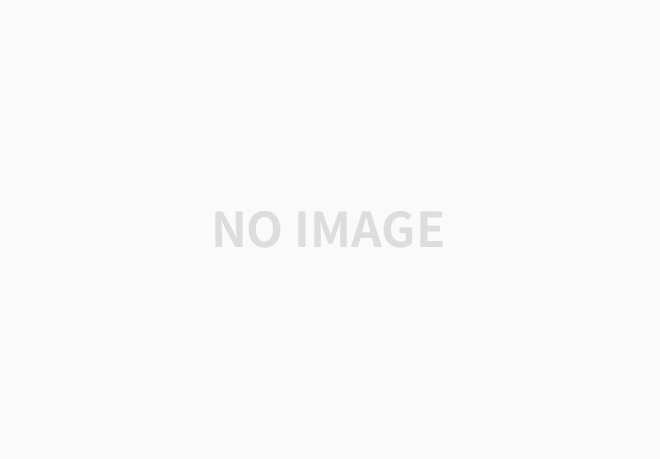
예제파일
send2.zip
0.00MB
참고 : 유닉스시스템 프로그래밍(한빛미디어)
'컴퓨터 기초 > 운영체제 실습' 카테고리의 다른 글
[운영체제 실습] 7.시스템 정보 다루기 - (로그인, 패스워드 정보) (0) | 2020.06.27 |
---|---|
[운영체제 실습] 6.파일과 디렉토리 (0) | 2020.06.25 |
[운영체제 실습] 4.에러처리 및 파일 다루기(low level) (0) | 2020.06.23 |
[운영체제 실습] 3.리눅스 커멘트라인 인자와 응용 (0) | 2020.06.22 |
[운영체제 실습] 2.파일 쓰기와 파일 읽기 (0) | 2020.06.16 |