iOS 배달앱 개발 예제(1) - (feat. CoreLocation, NSTimer, NSURLSession)
목표 : CoreLocation, NSTimer, NSURLSession 을 이용해서 배달앱의 핵심 기능인 위치정보를 얻어서 서버로 전송하는 예제를 구현해본다.
1.근태화면에서 출근 버튼을 누르면 현재 위치가 3초 간격으로 서버에 전송된다.
2.출동화면에서 출동 버튼을 누르면 현재 위치가 3초 간격으로 서버에 전송된다.
3.CoreLocation 라이브러리를 이용해서 싱글톤 객체로 만들어서 현재 위치를 가져온다.
4.NSURLSession 은 delegate 패턴을 이용해서 Get 메소드 호출이 성공했을때나 실패했을때 , Delegate 메소드가 호출되게 한다.
화면
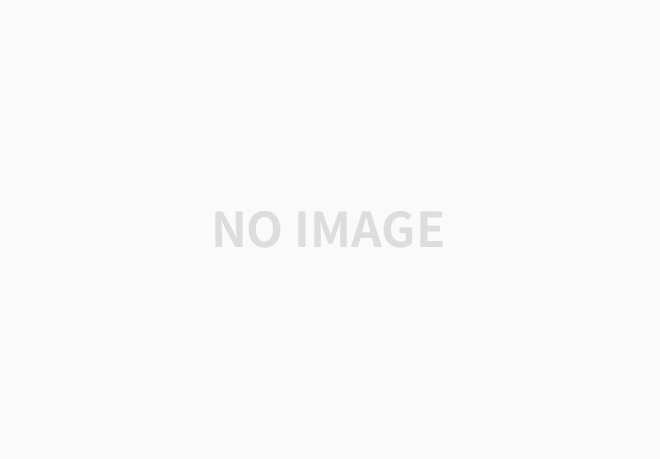
0.공통로직
CoreLocation 공통 로직 참고 - iOS CoreLocation 공통 모듈 - 싱글톤
NSURLSession 공통로직 참고 - NSURLSession 공통 모듈 예제
CoreLocation.zip
0.00MB
SessionOBJ.zip
0.00MB
1.근태관리 화면
근태관리화면에서 위의 공통 로직인 위치정보를 가져오는 클래스와, 통신하는 클래스를 import 해준다. 그리고 NSTimer를 이용해서 특정 시간 마다 위치 정보를 가져오는 함수를 호출해준다.
workVC.h
#import "CoreLocation.h"
#import <UIKit/UIKit.h>
#import "SessionObj.h"
NS_ASSUME_NONNULL_BEGIN
@interface workVC : UIViewController <MySessionDelegate>
{
NSTimer *timer;
}
//사용할 세션 객체
@property (strong,nonatomic) SessionObj *session;
//출근 / 퇴근 상태
@property (strong, nonatomic) IBOutlet UIBarButtonItem *workState;
//텍스트 뷰
@property (strong, nonatomic) IBOutlet UITextView *textViewForLocation;
@end
NS_ASSUME_NONNULL_END
workVC.m
출근 버튼 눌렀을때 goToWorkAction 메소드가 호출되면서 현재 위치가 3초 간격으로 텍스트뷰에 기록된다.
#import "workVC.h"
@interface workVC ()
@end
@implementation workVC
@synthesize workState, textViewForLocation;
- (void)viewDidLoad {
[super viewDidLoad];
NSLog(@"workVC viewDidLoad 진입");
//위치 정보 기록 텍스트 뷰 초기화
textViewForLocation.text = @"";
//NSURL Session 초기화
self.session = [[SessionObj alloc]init];
self.session.mySessionDelegate = self;
}
//출근 버튼 클릭시
- (IBAction)goToWorkAction:(id)sender {
NSLog(@"(근태) goToWorkAction 진입");
NSString *title = workState.title;
if ([title isEqualToString:@"출근"]) {
//참조 카운터 +
[CoreLocation sharedSingleton].refNum += 1;
//1,타이머 객체 생성
NSString *str = @"Timer";
timer = [NSTimer scheduledTimerWithTimeInterval:3.0
target:self
selector:@selector(updateMethod:)
userInfo:str
repeats:YES];
[workState setTitle:@"퇴근"];
}else{
[workState setTitle:@"출근"];
//타이머 종료
[timer invalidate];
//참조 카운터 -
[CoreLocation sharedSingleton].refNum -= 1;
NSInteger refNum = [[CoreLocation sharedSingleton] refNum];
NSLog(@"CoreLocation : 참조 카운터 : %d" , (int)refNum);
}
}
//타이머 업데이트 메소드
- (void) updateMethod:(NSTimer *)incomingTimer {
NSLog(@"(근태) Inside update method");
//위도
CLLocationDegrees latitude =
[[[[CoreLocation sharedSingleton] locationManager] location] coordinate].latitude;
//경도
CLLocationDegrees longitude =
[[[[CoreLocation sharedSingleton] locationManager] location] coordinate].longitude;
NSString *result = [NSString stringWithFormat:@"위도(lat) : %f , 경도(long) : %f \n" ,latitude ,longitude];
//텍스트뷰 위치 정보 갱신
textViewForLocation.text = [textViewForLocation.text stringByAppendingString:result];
//추후 실제 위도 경도 저장 url 로 변경!
NSURL *testurl = [NSURL URLWithString:@"https://reqres.in/api/users/7"];
[self.session startWithURL:testurl];
}
//통신 세션이 완료되면 호출되는 델리게이트 메소드 - - 결과에 따라 처리해줘야함
-(void)getResultDataFromMySessionData{
NSLog(@" (근태) getResultDataFromMySessionData viewDidLoad 메소드 진입");
NSLog(@"session.resultCode : %d" , self.session.resultCode);
NSLog(@"session.finishCode : %d" , self.session.finishCode);
NSString *resultString = [NSString stringWithFormat:@"%@", self.session.resultData];
NSLog(@"resultData : %@" , resultString);
}
@end
2.출동관리화면
moveVC.h
#import "CoreLocation.h"
#import <UIKit/UIKit.h>
#import "SessionObj.h"
NS_ASSUME_NONNULL_BEGIN
@interface moveVC : UIViewController <MySessionDelegate>
{
NSTimer *timer;
}
//사용할 세션 객체
@property (strong,nonatomic) SessionObj *session;
//출동 상태 / 출동 or 휴식
@property (strong, nonatomic) IBOutlet UIBarButtonItem *moveState;
//텍스트 뷰
@property (strong, nonatomic) IBOutlet UITextView *textViewForLocation;
@end
NS_ASSUME_NONNULL_END
moveVC.m
#import "moveVC.h"
@interface moveVC ()
@end
@implementation moveVC
@synthesize moveState, textViewForLocation;
- (void)viewDidLoad {
[super viewDidLoad];
NSLog(@"moveVC viewDidLoad 진입");
//NSURL Session 초기화
self.session = [[SessionObj alloc]init];
self.session.mySessionDelegate = self;
}
- (IBAction)moveAction:(id)sender {
NSLog(@"(출동) moveAction 진입");
NSString *title = moveState.title;
if ([title isEqualToString:@"출동"]) {
//참조 카운터 +
[CoreLocation sharedSingleton].refNum += 1;
//1,타이머 객체 생성
NSString *str = @"Timer";
timer = [NSTimer scheduledTimerWithTimeInterval:3.0
target:self
selector:@selector(updateMethod:)
userInfo:str
repeats:YES];
[moveState setTitle:@"종료"];
}else{
[moveState setTitle:@"출동"];
//타이머 종료
[timer invalidate];
//참조 카운터 -
[CoreLocation sharedSingleton].refNum -= 1;
NSInteger refNum = [[CoreLocation sharedSingleton] refNum];
NSLog(@"CoreLocation : 참조 카운터 : %d" , (int)refNum);
}
}
//타이머 업데이트 메소드
- (void) updateMethod:(NSTimer *)incomingTimer {
NSLog(@"(출동) Inside update method");
//위도
CLLocationDegrees latitude =
[[[[CoreLocation sharedSingleton] locationManager] location] coordinate].latitude;
//경도
CLLocationDegrees longitude =
[[[[CoreLocation sharedSingleton] locationManager] location] coordinate].longitude;
NSString *result = [NSString stringWithFormat:@"위도(lat) : %f , 경도(long) : %f \n" ,latitude ,longitude];
//텍스트뷰 위치 정보 갱신
textViewForLocation.text = [textViewForLocation.text stringByAppendingString:result];
//추후 실제 위도 경도 저장 url 로 변경!
NSURL *testurl = [NSURL URLWithString:@"https://reqres.in/api/users/8"];
[self.session startWithURL:testurl];
}
//통신 세션이 완료되면 호출되는 델리게이트 메소드 - 결과에 따라 처리해줘야함
-(void)getResultDataFromMySessionData{
NSLog(@"(출동) getResultDataFromMySessionData viewDidLoad 메소드 진입");
NSLog(@"session.resultCode : %d" , self.session.resultCode);
NSLog(@"session.finishCode : %d" , self.session.finishCode);
NSString *resultString = [NSString stringWithFormat:@"%@", self.session.resultData];
NSLog(@"resultData : %@" , resultString);
}
@end
결과
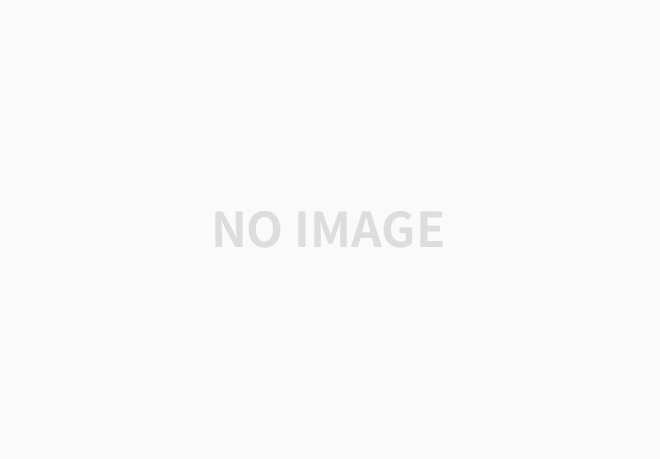
todoList...
1.실제 개발서버 구축 후 DB 생성해서 위치 정보 insert 하기
2.출퇴근 상태 데이터 , db에 저장, 출동, 종료 데이터 db에 저장 및 수정
3.위치정보 지도에 뿌려서 이동경로 그리기
참고
drive.google.com/file/d/10_kD5W-KhB3eGHhGxxnax29S1XBm48me/view?usp=sharing
'아이폰 개발 > ios 개념&튜토리얼' 카테고리의 다른 글
iOS Geofencing 예제 - 특정위치 안에 들어갔을때 알림(NSCoding, UserNotification) (1) | 2021.02.15 |
---|---|
iOS 배달앱 개발 예제(2) - (feat. 마커표시 MKAnnotation, CLLocationManagerDelegate, MKMapViewDelegate) (0) | 2021.02.15 |
iOS CoreLocation 공통 모듈 - 싱글톤 (0) | 2021.02.09 |
iOS 최상위뷰 체크 예제 - Curent RootViewController (0) | 2021.02.08 |
iOS 공통로그 & pch 파일 (0) | 2021.02.08 |